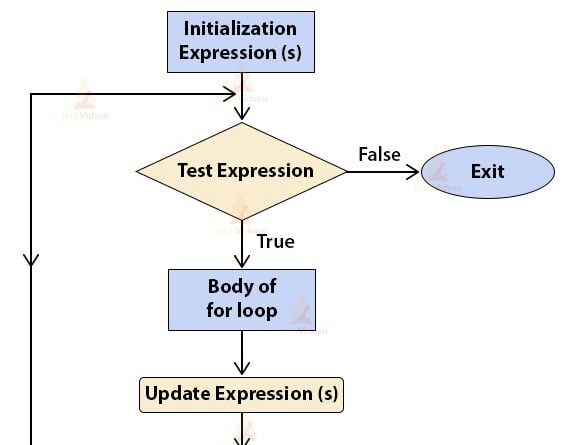
In this article, we’ll explore the basics of creating for loops in Java. You’ll learn what a for loop is, why it’s useful, and how to construct one in your Java code. By the end of this guide, you’ll have a solid understanding of how to use for loops to iterate through collections, perform repetitive tasks, and control your program flow in Java. Let’s get started!
Table of Contents
A Beginner’s Guide to Creating For Loops in Java
What is a for loop in Java?
A for loop is a fundamental construct in Java that allows you to repeatedly execute a block of code based on a specified condition. It is often used when you need to perform a certain task or iterate over a collection of elements. The for loop provides a concise and efficient way to control the flow of your program.
Definition of a for loop in Java
In Java, a for loop consists of three main components: initialization, condition, and update. The initialization is where you declare and initialize the loop control variable, which is typically an integer. The condition is a Boolean expression that is evaluated before each iteration of the loop. If the condition is true, the loop body is executed; otherwise, the loop terminates. The update is an expression that is evaluated at the end of each iteration, usually used to increment or decrement the loop control variable.
Purpose of using a for loop in Java
The main purpose of using a for loop in Java is to automate repetitive tasks or iterate over a sequence of elements. It allows you to write clean and concise code by encapsulating the repetitive logic within the loop body. By using a for loop, you can easily control the number of iterations and ensure that your code executes exactly as desired.
Syntax of a for loop in Java
The syntax of a for loop in Java is as follows:
for (initialization; condition; update) { // code to be executed }
Components of a for loop
The for loop consists of three components:
-
Initialization: This component is executed only once before the loop starts. It is used to initialize the loop control variable and is often used to set the initial value of the variable.
-
Condition: The condition is checked before each iteration of the loop. If the condition is true, the loop body is executed; otherwise, the loop terminates.
-
Update: The update component is executed at the end of each iteration. It is typically used to update the loop control variable, such as incrementing or decrementing its value.
Example of a basic for loop
Let’s say you want to print the numbers from 1 to 5. You can use a for loop to achieve this:
for (int i = 1; i <= 5; i++) { system.out.println(i); } < />ode>
In this example, the loop control variable i
is initialized to 1. The condition i <= 5< />ode> checks if
i
is less than or equal to 5. If the condition is true, the loop body System.out.println(i)
is executed, which prints the value of i
. After each iteration, the i
is incremented by 1 (i++
), and the process repeats until the condition becomes false.
Initializing variables in a for loop
What variables can be initialized in a for loop
In a for loop, you can initialize any variable of a compatible type. This includes primitive types such as int, char, and double, as well as reference types such as objects and arrays.
Examples of initializing variables in a for loop
Let’s consider a few examples to demonstrate the initialization of variables in a for loop:
- Initializing an integer variable:
for (int i = 0; i