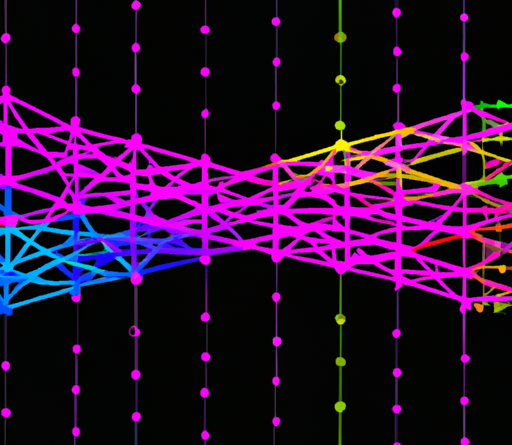
Welcome! In this article, you will learn the basics of for loops in Python. We will explore how these loops work and understand their purpose in programming. By the end, you will have a solid understanding of how to use for loops effectively in your Python code.
To begin, let’s dive into how for loops work in Python. A for loop is used to iterate over a sequence of elements, such as a list or a string. It allows you to perform a set of instructions repeatedly for each element in the sequence. By using a for loop, you can save time and effort by automating repetitive tasks in your code. Don’t worry if this concept seems a bit overwhelming at first – we’ll break it down step by step and provide examples to help you grasp the concept easily. So, let’s get started on this exciting journey of learning for loops in Python!
Table of Contents
Understanding the Basics of For Loops in Python
Introduction to For Loops
In Python, a for loop is a control flow statement that allows you to iterate over a sequence of elements, such as a list, string, or range of numbers. It executes a block of code repeatedly for each element in the sequence.
Purpose and Functionality of For Loops
The main purpose of a for loop is to automate repetitive tasks. It allows you to perform the same set of operations on each element in a sequence without having to write individual lines of code for each element.
Advantages of Using For Loops
Using for loops in your Python code offers several advantages. Firstly, it improves code readability by providing a concise and efficient way to iterate over sequences. Secondly, it simplifies the process of handling large amounts of data by allowing you to perform operations on each element in the sequence. Lastly, for loops are versatile and can be used in various scenarios, making them a powerful tool for writing efficient and scalable code.
Syntax of For Loops
Using Range Function
One common way to use a for loop is with the range function. This function generates a sequence of numbers that can be used to iterate over.
for i in range(start, stop, step): # code to be executed
In this syntax, start
is the starting value of the sequence, stop
is the ending (non-inclusive) value of the sequence, and step
is the increment value. The for loop will iterate over each number in the sequence, assigning it to the variable i
, and execute the code block.
Iterating Through a Sequence
Another way to use a for loop is by directly iterating through a sequence, such as a list or a string.
sequence = [1, 2, 3, 4, 5] for element in sequence: # code to be executed
In this example, the for loop will iterate over each element in the sequence
list, assigning it to the variable element
, and execute the code block.
Combining Multiple Sequences
You can also use multiple sequences in a for loop by combining them using the zip
function.
numbers = [1, 2, 3, 4, 5] letters = ['a', 'b', 'c', 'd', 'e'] for number, letter in zip(numbers, letters): # code to be executed
In this case, the for loop will iterate over both the numbers
and letters
sequences simultaneously, assigning each corresponding element to the variables number
and letter
, and execute the code block.
Using Enumerate Function
The enumerate
function is another useful tool when working with for loops. It allows you to iterate over a sequence while keeping track of the index of each element.
sequence = ['a', 'b', 'c', 'd', 'e'] for index, element in enumerate(sequence): # code to be executed
Here, the for loop will iterate over the sequence
list, assigning the index of each element to the variable index
and the element itself to the variable element
, and execute the code block.
Common Use Cases of For Loops
Iterating Over Lists
One of the most common use cases of for loops is iterating over lists. Let’s say you have a list of numbers and you want to perform a mathematical operation on each element.
numbers = [1, 2, 3, 4, 5] result = [] for number in numbers: result.append(number * 2) print(result) # Output: [2, 4, 6, 8, 10]
In this example, the for loop iterates over each element in the numbers
list, multiplies it by 2, and appends the result to the result
list.
Processing Data from Dictionaries
For loops are also useful for processing data from dictionaries. Let’s say you have a dictionary containing student names and their corresponding grades, and you want to calculate the average grade.
grades = {'John': 80, 'Alice': 90, 'Bob': 75, 'Jane': 85} total = 0 for grade in grades.values(): total += grade average = total / len(grades) print(average) # Output: 82.5
In this case, the for loop iterates over the values of the grades
dictionary, adds them to the total
variable, and then calculates the average by dividing the total by the number of grades.
Accessing Elements in a String
For loops can also be used to access individual characters in a string. Let’s say you have a string and you want to print each character on a separate line.
text = "Hello, World!" for char in text: print(char)
In this example, the for loop iterates over each character in the text
string and prints it to the console.
Performing Operations on Sets
For loops can also be used to perform operations on sets. Let’s say you have two sets of numbers and you want to find their intersection.
set1 = set2 = intersection = set() for element in set1: if element in set2: intersection.add(element) print(intersection) # Output:
In this case, the for loop iterates over each element in set1
and checks if it is also present in set2
. If it is, the element is added to the intersection
set.
Nested For Loops
Understanding Nested Loop Structure
Nested for loops are a powerful feature of Python that allow you to create patterns and perform complex iterations. A nested for loop is a loop inside another loop.
for i in range(5): for j in range(5): print(i, j)
In this example, the outer for loop iterates over the values 0 to 4, while the inner for loop also iterates over the values 0 to 4. The resulting output will be all possible combinations of i
and j
.
Creating Patterns with Nested For Loops
Nested for loops can be used to create patterns and shapes. Let’s say you want to print a triangle of asterisks.
for i in range(5): for j in range(i + 1): print('*', end='') print()
In this case, the outer for loop iterates over the values 0 to 4, while the inner for loop iterates over the values 0 to i
. The end=''
parameter in the print statement ensures that the asterisks are printed on the same line. The resulting output will be the following triangle:
* ** *** **** *****
Nested Loop Optimization Techniques
When working with nested for loops, it’s important to consider performance. Nested loops can be computationally expensive, especially when dealing with large data sets. Here are some optimization techniques to improve the efficiency of nested loops:
- Loop Reordering: Rearrange the loops to minimize the number of iterations and improve cache efficiency.
- Loop Unrolling: Unroll the inner loop by manually writing out the loop iterations. This can reduce the loop overhead and improve performance.
- Vectorization: Use vectorized operations or libraries like NumPy to perform computations on entire arrays instead of using nested loops.
Control Flow in For Loops
Using Break Statement
The break
statement allows you to exit a for loop prematurely. Let’s say you have a list of numbers and you want to find the first occurrence of a specific number.
numbers = [1, 2, 3, 4, 5] for number in numbers: if number == 3: break # exits the loop print(number) print("Loop finished")
In this example, when the for loop encounters the number 3, the break
statement is executed, and the loop terminates. Only the numbers 1 and 2 will be printed, and the message “Loop finished” will be displayed.
Using Continue Statement
The continue
statement allows you to skip the rest of the current iteration and move on to the next one. Let’s say you have a list of numbers and you want to skip the number 3.
numbers = [1, 2, 3, 4, 5] for number in numbers: if number == 3: continue # skip to the next iteration print(number) print("Loop finished")
In this case, when the for loop encounters the number 3, the continue
statement is executed, and the loop moves on to the next iteration. The number 3 will be skipped, and all other numbers will be printed. The message “Loop finished” will still be displayed at the end.
Skipping Iterations with Pass Statement
The pass
statement can be used to do nothing and acts as a placeholder. It is often used when creating a for loop structure that will be filled in later.
for i in range(5): if i