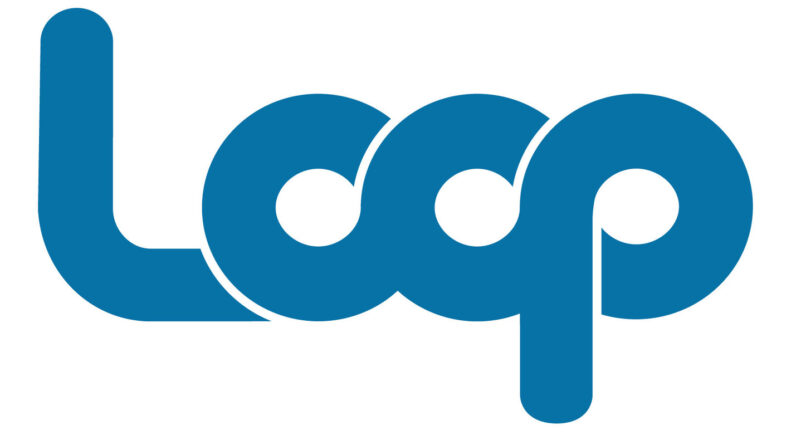
In this article, we will discuss how to create a for loop in Python. You will learn the basic syntax and structure of a for loop, as well as how to use it to iterate over a sequence of elements. By the end of this article, you will have a good understanding of how to implement for loops in your Python code. So let’s get started and explore the world of for loops in Python!
Table of Contents
Beginner’s Guide to Creating a For Loop in Python
If you are new to programming with Python, you may have heard about the concept of a for loop. A for loop is a powerful tool that allows you to iterate over a sequence of elements and perform a certain action for each element in the sequence. In this beginner’s guide, we will explore the basics of creating a for loop in Python, its syntax, and various ways you can use it in your code.
What is a For Loop?
A for loop is a control structure in Python that iterates over a sequence of items. It allows you to perform a specific action for each item in the sequence, such as executing a block of code or modifying the elements. With the help of a for loop, you can automate repetitive tasks and process large amounts of data efficiently.
Syntax of a For Loop
The syntax of a for loop in Python is quite simple. It consists of the keywords for
and in
, followed by a variable that represents each element in the sequence, and the sequence itself. Here’s how a basic for loop is structured:
for variable in sequence: # code to be executed for each element in the sequence
The variable
represents the current element in the sequence, and you can choose any valid variable name. The sequence
can be a list, tuple, string, or any other iterable object in Python.
Why Use For Loops in Python?
Before we dive deeper into the details of creating a for loop, let’s explore why you might want to use for loops in your Python code.
Benefits of Using For Loops
One of the main benefits of using for loops is that they allow you to iterate over a sequence of elements without the need for manual indexing. This makes your code more readable, as you can focus on the logic of your program rather than managing index values.
For loops are also highly versatile and flexible. They can be used to perform a wide range of tasks, such as calculating the sum of all numbers in a list, finding specific elements in a sequence, or even creating new sequences based on existing ones. By leveraging the power and simplicity of for loops, you can write more efficient and concise code.
Comparison to Other Looping Structures
In Python, there are different looping structures available, such as while loops and do-while loops. While loops are generally used when you want to repeat a specific block of code as long as a certain condition is true. Do-while loops, on the other hand, execute the block of code at least once before checking the condition.
Compared to these looping structures, for loops are best suited when you know the number of iterations in advance or want to iterate over a fixed sequence. They provide an intuitive and straightforward way to loop through elements, making them ideal for many programming scenarios.
Creating a Basic For Loop
Now that you understand the basics of for loops and their benefits, let’s dive into creating a basic for loop step by step.
Initializing the Loop Variable
To start a for loop, you need to initialize the loop variable with the first element of the sequence. The loop variable represents the current element in each iteration of the loop. Here’s an example:
fruits = ['apple', 'banana', 'cherry'] for fruit in fruits: # code to be executed for each fruit
In this example, the loop variable fruit
is initialized with the first element of the fruits
list, which is 'apple'
.
Defining the Loop Condition
After initializing the loop variable, the for loop continues until it reaches the last element of the sequence. The loop condition is automatically managed by the for loop itself, so you don’t need to worry about incrementing or decrementing the loop variable manually.
Iterating through a Sequence
During each iteration of the for loop, the loop variable takes on the value of the current element in the sequence. You can then perform any desired action or execute a block of code for each element. For example, let’s print each fruit in the fruits
list:
fruits = ['apple', 'banana', 'cherry'] for fruit in fruits: print(fruit)
This code will output:
apple banana cherry
Body of the Loop
The body of the for loop consists of the code that is executed for each element in the sequence. It is indented to signify that it belongs to the loop. You can include any valid Python code within the loop, such as calculations, conditionals, or function calls.
Updating the Loop Variable
After each iteration, the loop variable is automatically updated to the next element in the sequence. This continues until the for loop reaches the end of the sequence. You don’t need to update the loop variable manually; the for loop takes care of it for you.
Using Range() Function with For Loop
The range()
function is a built-in function in Python that generates a sequence of numbers. This function is commonly used in conjunction with for loops to specify the number of iterations or to iterate over a sequence of numbers.
Understanding the Range() Function
The range()
function takes up to three arguments: start
, stop
, and step
. By default, the start
argument is 0, and the step
argument is 1. The stop
argument specifies the number at which the sequence stops (exclusive).
Using Range() to Specify the Number of Iterations
One common usage of the range()
function with for loops is to specify the number of iterations. For example, to iterate five times, you can use range(5)
:
for i in range(5): # code to be executed for each iteration
This will iterate five times, with the loop variable i
taking the values 0, 1, 2, 3, and 4.
Using Range() to Iterate over a Sequence
Another way to utilize the range()
function is to iterate over a sequence of numbers. Suppose you have a list of numbers and want to perform an action for each element. You can use the range(len(sequence))
construct:
numbers = [1, 2, 3, 4, 5] for i in range(len(numbers)): print(numbers[i])
This code will output:
1 2 3 4 5
In this example, the range of len(numbers)
generates the sequence of numbers 0, 1, 2, 3, 4
, which corresponds to the indices of the numbers
list.
Nested For Loops
In addition to basic for loops, Python also supports nested for loops. A nested for loop is a loop inside another loop and is used to iterate over multiple sequences simultaneously or perform repetitive actions in a hierarchical manner.
Definition and Purpose of Nested For Loops
Nested for loops are useful when you need to iterate over multiple sequences or perform repeated actions in a nested structure. For example, when working with a two-dimensional list or matrix, you can use nested for loops to access each element individually.
Syntax for Nested For Loops
The syntax for nested for loops is similar to basic for loops, but with an additional level of indentation for each inner loop. Here’s an example of a nested for loop:
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] for row in matrix: for element in row: # code to be executed for each element in the matrix
In this example, the outer loop iterates over each row in the matrix, while the inner loop iterates over each element in the current row.
Examples of Nested For Loops
Nested for loops can be used in various scenarios, such as generating combinations, implementing matrix operations, or processing hierarchical data structures. Let’s consider an example of calculating the sum of numbers in a two-dimensional list:
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] sum = 0 for row in matrix: for element in row: sum += element print(sum)
This code will output:
45
In this example, the nested for loops iterate through each element in the matrix and accumulate their sum in the sum
variable.
Break and Continue Statements
Python provides two control statements, break
and continue
, that allow you to alter the flow of a for loop according to specific conditions.
Using the Break Statement to Exit a Loop
The break
statement is used to prematurely exit a loop when a certain condition is met. You can use this statement to terminate the loop before it reaches the last element or based on any other desired condition.
For example, let’s find the first negative number in a list and exit the loop once we find it:
numbers = [1, 2, -3, 4, -5, 6] for number in numbers: if number