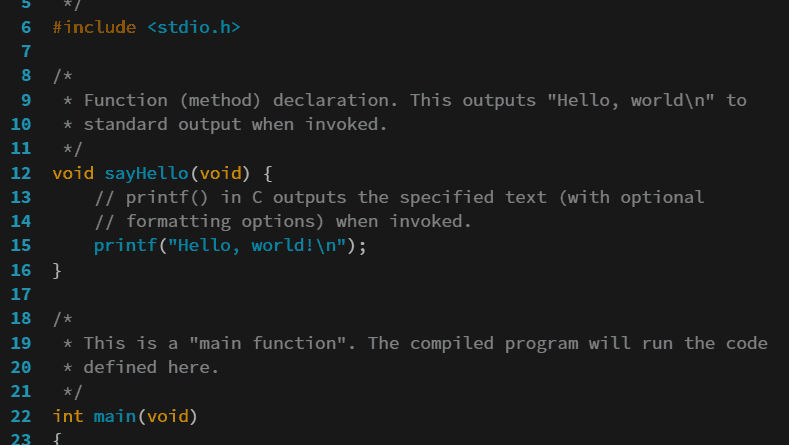
Have you ever wondered what the create command is in programming? It’s a commonly used command that allows you to create something new or initialize a new instance of an object. In programming, creating something refers to the process of defining and setting up a new component, whether it’s a variable, a function, or even an entire program. With the create command, you have the power to generate new elements and bring them to life in your code.
In this article, we’ll dive deeper into the create command and explore its various applications in programming. You’ll learn how it can be used to create different types of objects, such as arrays, strings, and classes. We’ll also discuss the importance of proper initialization and how the create command plays a crucial role in ensuring that your code runs smoothly. So, if you’re ready to expand your programming knowledge and harness the power of the create command, keep reading to find out more!
Table of Contents
What is the Create Command in Programming
The Create command is a fundamental aspect of programming that allows developers to create new instances of objects, structures, or entities within their code. It serves as a means to initialize and allocate necessary resources for these newly created elements. The Create command is widely utilized across various programming languages and paradigms, and understanding its syntax and usage is essential for effectively utilizing it in programming tasks.
Definition of the Create Command
In programming, the Create command refers to the action of creating a new instance or object. It involves allocating memory and setting initial values or properties for the newly created entity. The Create command provides developers with the ability to dynamically generate these instances within their programs, offering flexibility and adaptability in solving complex problems.
Purpose of the Create Command
The primary purpose of the Create command is to enable developers to generate new objects, structures, or entities that are required to fulfill specific tasks within a program. By creating new instances, programmers can model and manipulate data, perform operations, and implement algorithms efficiently. The Create command is instrumental in managing memory, initializing variables, and defining the state of objects, ultimately contributing to the overall functionality of a program.
Common Programming Languages that Use the Create Command
The Create command is a ubiquitous feature across numerous programming languages. Many high-level programming languages provide built-in functions or keywords to facilitate the creation of new instances. Here are some commonly used programming languages that utilize the Create command:
-
Java: In Java, the
new
keyword is used to create new instances of objects. It invokes the class constructor and returns a reference to the newly created object. -
Python: Python uses the
class
keyword along with a constructor method named__init__()
to create new instances of an object. Python also supports using functions to create objects. -
C#: C# includes the
new
keyword to create new instances of classes. It invokes the constructor method of the class and returns an object of that type. -
JavaScript: JavaScript uses the
new
keyword to create new instances of objects. Thenew
keyword calls the constructor method defined within the object.
These are just a few examples, but the Create command is widely applicable across an array of programming languages and frameworks.
Syntax and Usage of the Create Command
Understanding the syntax and usage of the Create command is crucial for effectively utilizing it in programming tasks. The following sections will provide an overview of the basic syntax, parameters, and arguments, as well as examples of using the Create command in practice.
Basic Syntax of the Create Command
The syntax for the Create command may vary depending on the programming language, but it typically follows a pattern. Here is a general representation of the syntax:
new ClassName(arguments)
In this syntax, new
is the keyword used to create a new instance, ClassName
refers to the name of the class or object being instantiated, and arguments
represent any parameters or values required by the constructor method.
Parameters and Arguments in the Create Command
The Create command often involves passing parameters or arguments to the constructor method of the class or object being created. These parameters provide initial values or configurations for the newly created instance. The number and type of arguments required depend on the implementation of the constructor method. It is essential to consult the documentation or refer to the programming language’s guidelines to determine the specific parameters and their corresponding data types for the Create command.
Examples of Using the Create Command
To illustrate the usage of the Create command, let’s consider a simple example in Java. Suppose we have a class called Person
with attributes such as name and age. We can create new instances of the Person
class using the following code:
Person person1 = new Person("John Doe", 25); Person person2 = new Person("Jane Smith", 30);
In this example, the new
keyword is used to create two new instances of the Person
class, person1
and person2
. The arguments "John Doe", 25
and "Jane Smith", 30
represent the initial values for the name
and age
attributes of the Person
objects.
This is just a basic example, but it showcases the fundamental usage of the Create command in instantiating objects within a program.
Understanding the Create Command in Different Programming Paradigms
The usage and implementation of the Create command can vary across different programming paradigms. Let’s explore how the Create command is utilized in procedural programming, object-oriented programming, and functional programming paradigms.
Procedural Programming
In procedural programming, the Create command is commonly used to create and manipulate data structures or variables. It involves allocating memory and initializing variables to hold data.
For example, in C, the malloc()
function is used to dynamically allocate memory for a variable:
int* array = malloc(size * sizeof(int));
In this example, the malloc()
function is utilized to create a dynamically allocated array of integers.
Object-Oriented Programming
In object-oriented programming (OOP), the Create command is central to creating objects from classes. It allows programmers to define the properties and behaviors of objects through the use of classes and their corresponding constructors.
For instance, in C++, the Create command is commonly used as follows:
class Person { public: string name; int age; Person(string n, int a) { name = n; age = a; } }; int main() { Person person1("John Doe", 25); Person person2("Jane Smith", 30); return 0; }
In this example, the Person
class defines the properties name
and age
, along with a constructor that initializes these properties. The Create command is used within the main()
function to instantiate two Person
objects, person1
and person2
.
Functional Programming
Functional programming focuses on computations and transformations of data rather than objects and classes. While the concept of creating instances might be less prominent in functional programming, the Create command can still be relevant in certain scenarios.
For example, in Haskell, a functional programming language, records can be used to define data structures. The Create command can be used to create instances of these records:
data Person = Person { name :: String, age :: Int } person1 = Person { name = "John Doe", age = 25 } person2 = Person { name = "Jane Smith", age = 30 }
In this example, the Person
record is defined with a name
and age
field. The Create command is used to create two instances of the Person
record, person1
and person2
.
Understanding how the Create command is used within different programming paradigms allows developers to effectively leverage its benefits in various contexts.
Key Concepts Related to the Create Command
The Create command is closely linked to several key concepts in programming. Let’s explore these concepts and their relationship with the Create command.
Data Structures and the Create Command
Data structures play a vital role in organizing and storing data efficiently. The Create command is often used to create instances of data structures such as arrays, lists, or trees. By allocating memory and initializing these data structures, developers can utilize them to store and manipulate data effectively.
Memory Management and the Create Command
Memory management is crucial in programming to prevent memory leaks, optimize performance, and ensure the efficient utilization of system resources. The Create command is responsible for allocating memory for newly created instances. Equally important is the responsible deallocation of memory through appropriate techniques such as garbage collection or manual memory management, depending on the programming language.
Instantiate and the Create Command
The terms “instantiate” and “create” are often used interchangeably in the context of programming. Both refer to the process of generating a new instance of an object or class. The Create command is the mechanism through which the object or class is instantiated, providing developers with the ability to utilize and manipulate these instances within their programs.
Best Practices for Using the Create Command
To maximize the potential benefits of the Create command, developers should adhere to certain best practices. Here are a few key practices to keep in mind when using the Create command:
Encapsulation and the Create Command
Encapsulation is an essential principle in object-oriented programming. It involves encapsulating data and methods within a class, controlling access to these components through appropriate class members. When using the Create command, it is good practice to encapsulate the creation logic within a class or factory method, ensuring that the process of creating instances remains encapsulated and modular.
Error Handling and the Create Command
Error handling is crucial for robust and reliable software. When creating instances, it is necessary to handle potential errors that may occur during the creation process. Whether it’s checking for invalid inputs, memory allocation failures, or other exceptional situations, incorporating proper error handling mechanisms enhances the reliability and stability of programs.
Optimizing Performance with the Create Command
The Create command involves memory allocation and initialization, which can impact the performance of a program, especially when creating a large number of instances. It is important to optimize performance by minimizing unnecessary duplicate creations, utilizing memory efficiently, and considering alternative data structures or caching mechanisms when appropriate.
Potential Challenges and Pitfalls with the Create Command
While the Create command is a powerful tool, there are potential challenges and pitfalls that developers should be aware of. Here are a few common issues to watch out for:
Memory Leaks
Improper memory management can lead to memory leaks, where allocated memory is not released, causing memory usage to increase over time. It is essential to ensure proper cleanup and deallocation of memory to avoid such leaks when using the Create command.
Resource Overuse
Creating excessive instances or objects can lead to resource overuse, such as excessive memory consumption or CPU load. It is important to analyze and optimize resource usage when utilizing the Create command to avoid unnecessary strain on system resources.
Inefficient Code Execution
Inefficient use of the Create command can result in poor performance and slower execution times. For example, creating new instances unnecessarily in performance-critical sections of code can impact the overall efficiency of a program. It is important to review and analyze the usage of the Create command to identify potential bottlenecks and optimize code execution when required.
Alternatives to the Create Command
Although the Create command is widely used, depending on the programming language or specific requirements, alternative approaches may be preferred. Here are a few alternative commands or techniques that can be used instead of the Create command:
Copy Command
In certain scenarios, it may be more appropriate to create new instances by copying existing ones rather than utilizing the Create command. Copy commands allow developers to duplicate existing objects, structures, or entities, preserving their values and state.
Instantiate Command
While the Create command is most commonly associated with object-oriented programming, certain programming languages or frameworks may utilize the term “instantiate” rather than “create” when referring to the process of generating new instances.
Generate Command
In some programming languages, the term “generate” may be used as an alternative to the Create command. It implies the creation of new instances or data structures based on specific rules or algorithms.
Examples of the Create Command in Real-World Applications
The Create command finds extensive application in various real-world scenarios. Here are a few examples of how the Create command is utilized in practical applications:
Database Creation
In database management systems, the Create command is used to create new databases, tables, or other database objects. By utilizing the Create command, developers can define the structure and schema of databases, enabling efficient storage and retrieval of data.
File System Creation
File systems rely on the Create command for creating directories, files, or other filesystem components. The Create command allows for the creation of new files or directories, setting their initial attributes and permissions.
Graphical User Interface Creation
Graphical user interface (GUI) frameworks often utilize the Create command extensively. It enables developers to create the various user interface components, such as buttons, windows, or input fields. The Create command is fundamental in GUI development, allowing for the instantiation of these components and their subsequent manipulation.
Future Trends and Developments in the Create Command
As programming languages and frameworks continue to evolve, the Create command is likely to undergo further advancements and improvements. Here are some potential future trends and developments to watch for:
Automation and the Create Command
Automation capabilities may be integrated into the Create command, allowing for the more efficient creation and management of instances. Automated routines or smart algorithms could aid in optimizing the creation process based on specific requirements or patterns.
Integration with Artificial Intelligence
The advancement of artificial intelligence (AI) technologies may lead to the integration of intelligent algorithms within the Create command. AI-powered assistants or auto-generators could provide suggestions or automate the creation of complex instances, easing the programming process.
Increased Customization Options
Enhancements to the Create command may introduce more customization options, enabling developers to tailor the creation process to specific needs. This could involve defining additional parameters or configuring advanced settings for the initialization of instances.
Conclusion
The Create command is a fundamental aspect of programming, allowing developers to dynamically generate new instances of objects, structures, or entities within their programs. By understanding its syntax, usage, and importance in different programming paradigms, developers can effectively utilize the Create command to model data, perform operations, and implement algorithms efficiently.
While the Create command offers immense power and flexibility, developers must be mindful of potential challenges and pitfalls. Proper memory management, error handling, and optimization of code execution are crucial for leveraging the benefits of the Create command effectively.
As programming languages and frameworks evolve, the Create command is likely to witness further advancements and integration with emerging technologies. Automation, AI integration, and increased customization options are some potential future trends that may enhance the capabilities of the Create command, making programming even more efficient and versatile.